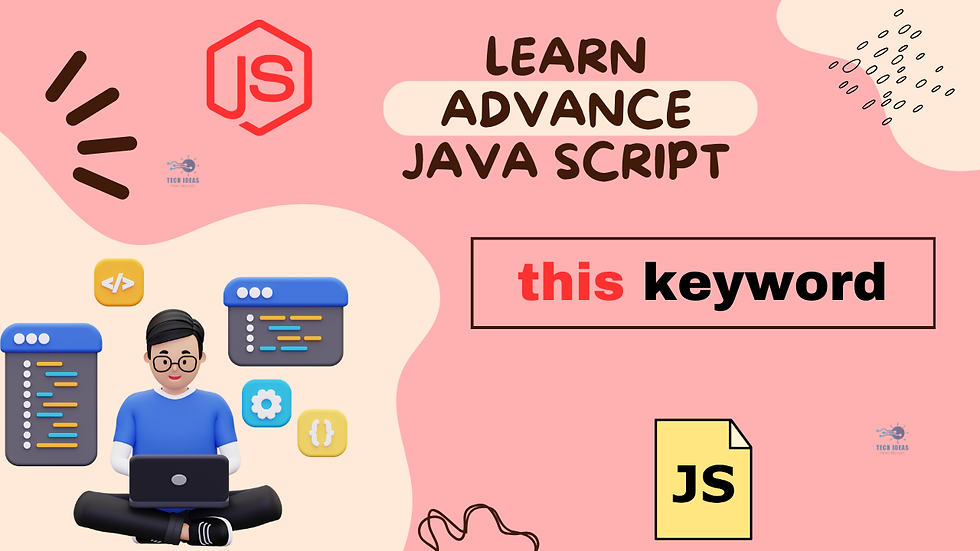
The 'this' keyword in JavaScript is a powerful yet often misunderstood concept. It plays a crucial role in how functions and objects interact with each other. In this blog post, we'll break down what 'this' means, how it behaves in different contexts, and provide practical examples to clarify its use.
Understanding the this Keyword in JavaScript
What is 'this' in JavaScript?
The 'this' keyword in JavaScript refers to the object that is currently executing a function. However, what 'this' points to can change depending on the context in which a function is called. Understanding this behavior is key to mastering JavaScript's object-oriented nature.
In general, 'this' is used to reference the context in which the function is executed. The context can vary depending on whether the function is called as a method, constructor, or in the global scope.
Key Concepts of 'this'
Global Context
Object Method
Constructor Function
Arrow Functions
Explicit Binding (call, apply, bind)
Let's explore each concept in detail.
1. 'this' in the Global Context
In the global context, the value of 'this' refers to the global object. In browsers, the global object is 'window', while in Node.js, it’s 'global'.
Example:
console.log(this); // In the browser, this will log the window object.
If 'this' is used inside a regular function that is not part of an object, it also refers to the global object.
function showThis() {
console.log(this);
}
showThis(); // In the browser, this will also log the window object.
2. 'this' in Object Methods
When a function is called as a method of an object, 'this' refers to the object the method belongs to.
Example:
const person = {
name: 'John',
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
person.greet(); // Output: Hello, my name is John
Here, 'this.name' refers to the 'name' property of the 'person' object.
3. 'this' in Constructor Functions
When a function is used as a constructor (with the 'new' keyword), 'this' refers to the newly created object.
Example:
function Person(name) {
this.name = name;
}
const john = new Person('John');
console.log(john.name); // Output: John
In this case, 'this' inside the 'Person' constructor refers to the new object that is created when 'new Person('John')' is called.
4. 'this' in Arrow Functions
Arrow functions handle 'this' differently than regular functions. Instead of binding 'this' to the object that calls the function, arrow functions capture the value of 'this' from the surrounding lexical context (i.e., where the arrow function was defined).
Example:
const person = {
name: 'John',
greet: () => {
console.log(`Hello, my name is ${this.name}`);
}
};
person.greet(); // Output: Hello, my name is undefined
Here, 'this.name' does not work as expected because arrow functions do not have their own 'this'. They inherit 'this' from their parent scope. In this case, the 'this' inside 'greet()' refers to the global object, not the 'person' object.
5. Explicit Binding: 'call', 'apply', and 'bind'
JavaScript provides ways to explicitly bind 'this' to a specific value using 'call()', 'apply()', and 'bind()' methods.
'call()': Invokes a function with a specific 'this' value.
'apply()': Similar to 'call()', but arguments are passed as an array.
'bind()': Returns a new function with a specific 'this' value.
Example with 'call()':
const person = {
name: 'John',
};
function greet() {
console.log(`Hello, my name is ${this.name}`);
}
greet.call(person); // Output: Hello, my name is John
Example with 'bind()':
const greetPerson = greet.bind(person);
greetPerson(); // Output: Hello, my name is John
Practical Examples of Using 'this'
Object-Oriented Programming: 'this' is used extensively in classes and objects to refer to properties and methods of the current object.
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
displayInfo() {
console.log(`Car: ${this.make} ${this.model}`);
}
}
const car1 = new Car('Toyota', 'Camry');
car1.displayInfo(); // Output: Car: Toyota Camry
Event Handlers in the DOM: When an event handler is invoked, 'this' refers to the element that triggered the event.
document.querySelector('button').addEventListener('click', function() {
console.log(this); // Refers to the button element
});
Comments