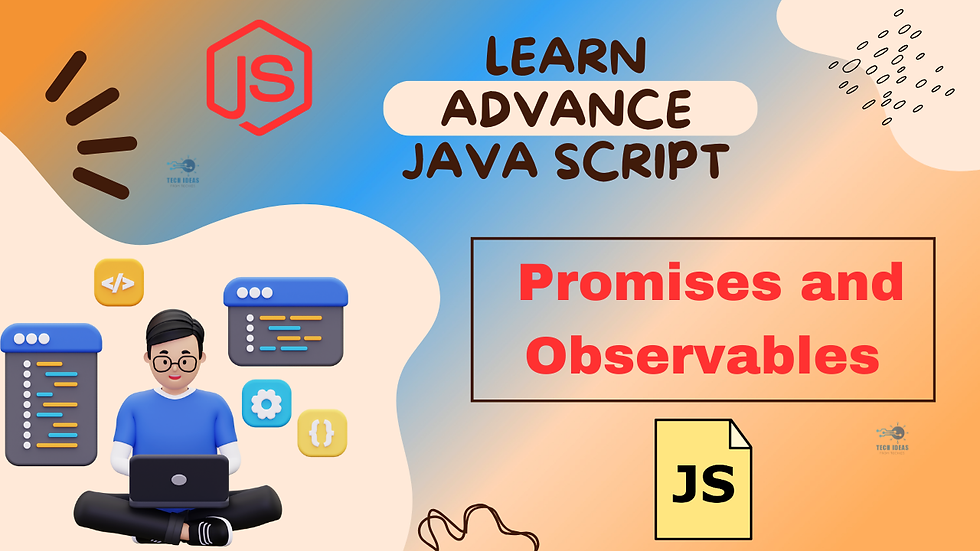
JavaScript is a powerful language for handling asynchronous operations, and two key concepts that help in managing these operations are Promises and Observables. Both are used to handle asynchronous tasks, but they do so in different ways. In this blog post, we'll explore what Promises and Observables are, how they work, and provide examples to help you understand them better.
Understanding Promises and Observables in JavaScript
1. What Are Promises?
Promises in JavaScript are a way to handle asynchronous operations, such as API calls or file handling. They represent a value that might not be available yet but will be resolved in the future.
A Promise can be in one of three states:
Pending: The initial state, neither fulfilled nor rejected.
Fulfilled: The operation was completed successfully, and the promise has a value.
Rejected: The operation failed, and the promise has a reason for failure (an error).
Example of a Promise:
let myPromise = new Promise(function(resolve, reject) {
let success = true; // Simulate an operation
if (success) {
resolve("Operation succeeded!");
} else {
reject("Operation failed!");
}
});
myPromise
.then(function(value) {
console.log(value); // Output: Operation succeeded!
})
.catch(function(error) {
console.log(error);
});
In this example, the myPromise is either resolved or rejected based on the success of the operation. The .then() method handles the resolved value, while .catch() handles any errors.
Using Promises in Real-Life Scenarios:
Promises are often used when dealing with APIs, for example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Here, fetch returns a promise that resolves with the response data if successful, or an error if the request fails.
2. What Are Observables?
Observables are a more powerful and flexible way to handle asynchronous operations, particularly in complex scenarios like event handling, multiple API calls, or real-time data streams.
An Observable emits multiple values over time, unlike Promises, which handle a single value or event. Observables are often used in reactive programming to handle sequences of asynchronous events.
Example of an Observable:
import { Observable } from 'rxjs';
let myObservable = new Observable((observer) => {
observer.next('First value');
observer.next('Second value');
setTimeout(() => {
observer.next('Third value');
observer.complete();
}, 2000);
});
myObservable.subscribe({
next(value) {
console.log(value);
},
complete() {
console.log('Observable completed');
}
});
In this example, the myObservable emits multiple values over time. The observer subscribes to these values and processes them as they are emitted. The Observable also has a complete method that signals when it’s done emitting values.
Using Observables in Real-Life Scenarios:
Observables are commonly used in frameworks like Angular, which utilizes the RxJS library to handle HTTP requests, user interactions, and more:
import { of } from 'rxjs';
import { map } from 'rxjs/operators';
of(1, 2, 3)
.pipe(map(value => value * 2))
.subscribe(value => console.log(value)); // Output: 2, 4, 6
This example demonstrates the power of Observables with operators like map to transform emitted values before they are handled by the subscriber.
3. Key Differences Between Promises and Observables
Single vs. Multiple Values:
Promises handle a single value or event.
Observables can handle multiple values over time.
Lazy vs. Eager:
Promises are eager, meaning they start executing immediately when created.
Observables are lazy, meaning they don’t start emitting values until you subscribe to them.
Cancellation:
Promises cannot be canceled once started.
Observables can be unsubscribed from, effectively canceling the operation.
Operators:
Observables come with a rich set of operators (e.g., map, filter, merge) for handling sequences of values, making them more flexible for complex scenarios.
4. When to Use Promises vs. Observables
Use Promises when you need to handle a single asynchronous event, such as fetching data from an API.
Use Observables when dealing with multiple values or events, such as user interactions, real-time data streams, or when you need more advanced features like cancellation and chaining operations.
Комментарии