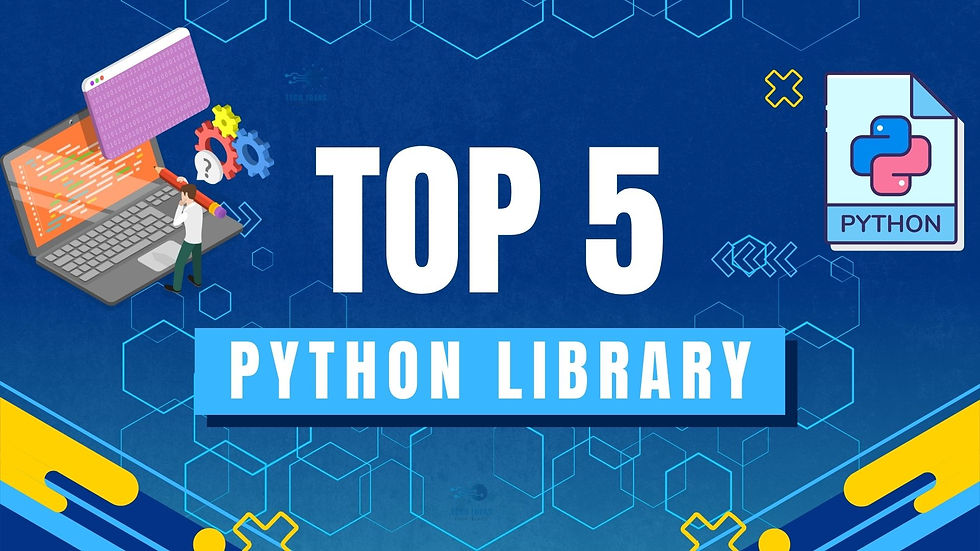
Python is a popular programming language known for its simplicity and versatility. One of the reasons for its success is the availability of a vast collection of libraries that make various tasks easier. In this blog, we’ll explore the top 5 most useful Python libraries that you should know, along with their key features and use cases.
1. NumPy
Purpose: Numerical Computations
NumPy (Numerical Python) is a fundamental library for scientific computing in Python. It provides support for working with arrays and matrices, along with a wide range of mathematical functions to operate on them.
Key Features:
Efficient handling of large datasets with multi-dimensional arrays.
Mathematical functions such as trigonometric, statistical, and algebraic operations.
Integration with other libraries like Pandas and Matplotlib.
Use Cases:
Data analysis and manipulation.
Performing complex mathematical operations on arrays.
Machine learning algorithms that require numerical data processing.
Example:
import numpy as np
array = np.array([1, 2, 3, 4])
print(np.mean(array)) # Output: 2.5
2. Pandas
Purpose: Data Manipulation and Analysis
Pandas is an essential library for data manipulation and analysis in Python. It provides easy-to-use data structures like DataFrames, which are powerful tools for working with structured data. With Pandas, you can load, manipulate, and analyze data from various sources such as CSV files, Excel sheets, or SQL databases.
Key Features:
Data structures like Series (1-dimensional) and DataFrames (2-dimensional).
Handling missing data, filtering, and merging datasets.
Time series analysis and data transformation capabilities.
Use Cases:
Data preprocessing for machine learning models.
Analyzing financial data or sales data.
Working with large datasets in an organized and efficient way.
Example:
import pandas as pd
data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]}
df = pd.DataFrame(data)
print(df)
3. Matplotlib
Purpose: Data Visualization
Matplotlib is one of the most widely used libraries for creating static, animated, and interactive visualizations in Python. It is highly flexible, allowing you to create a wide variety of graphs and plots to represent your data visually.
Key Features:
Supports a variety of plots like line charts, bar charts, scatter plots, and histograms.
Highly customizable for creating complex visualizations.
Compatible with other Python libraries such as NumPy and Pandas.
Use Cases:
Visualizing data trends over time.
Creating publication-quality graphs and charts.
Exploratory data analysis in data science projects.
Example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.xlabel('X axis')
plt.ylabel('Y axis')
plt.show()
4. Requests
Purpose: HTTP Requests Handling
Requests is a user-friendly library that simplifies sending HTTP requests in Python. Whether you’re consuming web APIs, fetching data from the internet, or interacting with web services, Requests makes it straightforward and easy to manage.
Key Features:
Simple API for making HTTP requests (GET, POST, PUT, DELETE).
Handling JSON responses easily.
Managing sessions, cookies, and authentication.
Use Cases:
Consuming web services and RESTful APIs.
Automating web interactions.
Downloading and uploading files from/to the web.
Example:
import requests
response = requests.get('https://api.github.com')
print(response.json())
5. TensorFlow
Purpose: Machine Learning and Deep Learning
TensorFlow is a comprehensive open-source platform for machine learning and artificial intelligence. Developed by Google, it is widely used for building deep learning models and neural networks. TensorFlow provides an easy-to-use interface for creating, training, and deploying machine learning models at scale.
Key Features:
Supports deep learning, neural networks, and other machine learning models.
Optimized for both CPU and GPU performance.
Includes tools for model deployment and serving.
Use Cases:
Image recognition and processing.
Natural language processing (NLP).
Creating recommendation systems, chatbots, and predictive models.
Example:
import tensorflow as tf
model = tf.keras.Sequential([tf.keras.layers.Dense(units=1, input_shape=[1])])
model.compile(optimizer='sgd', loss='mean_squared_error')
These top 5 Python libraries are essential tools for various tasks, ranging from data analysis and visualization to machine learning and web scraping. NumPy and Pandas make working with data easier, Matplotlib helps you visualize it, Requests simplifies web interactions, and TensorFlow allows you to build powerful AI models. Whether you're a beginner or an experienced developer, these libraries will significantly enhance your Python programming skills.
Comments