Understanding and Solving Undefined or Null References in JavaScript
- prabuganesan
- Aug 24, 2024
- 4 min read
Understanding and Solving Undefined or Null References in JavaScript
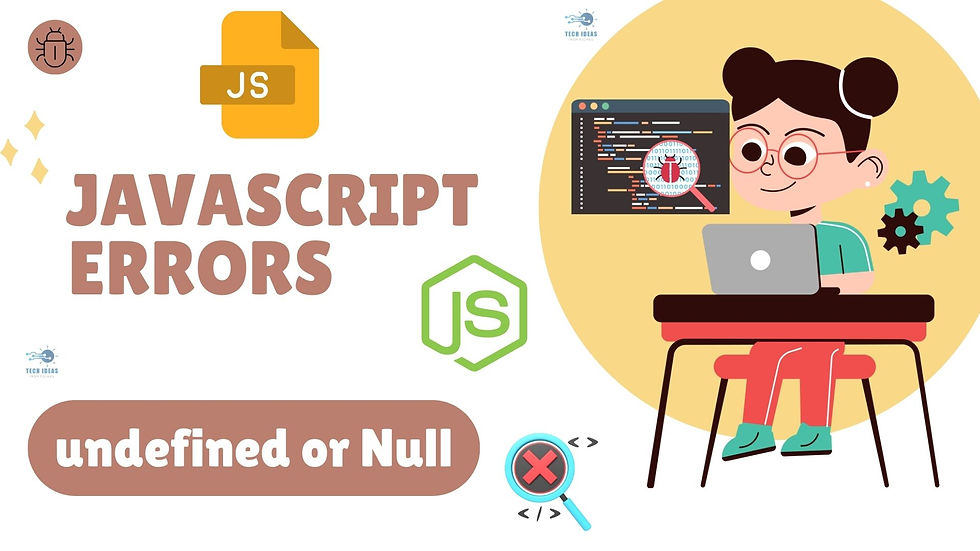
In JavaScript, one of the most common errors developers encounter is trying to access or manipulate variables that are either `undefined` or `null`. These errors can lead to broken functionality, confusing bugs, and application crashes if not handled properly. In this blog post, we’ll explore what `undefined` and `null` mean, why reference errors occur, and how to handle them effectively.
What are `undefined` and `null`?
In JavaScript, both `undefined` and `null` are used to represent the absence of a value, but they have distinct meanings and uses:
undefined: This is the default value of a variable that has been declared but not assigned a value. It also represents the absence of a property or index in objects or arrays, respectively.
let x; // x is undefined
console.log(x); // Output: undefined
null: This is an assignment value that represents the intentional absence of any object value. It is often used to reset or clear a value manually.
let obj = null; // obj is explicitly set to null
Both `undefined` and `null` are primitive data types in JavaScript, and understanding how they work is essential to avoiding common errors.
Common Scenarios Leading to Undefined or Null References
1. Accessing Properties of `undefined` or `null`
A frequent error occurs when attempting to access a property or call a method on a variable that is either `undefined` or `null`.
Example:
let person;
console.log(person.name); // TypeError: Cannot read property 'name' of undefined
Here, the variable `person` is `undefined`, and attempting to access the `name` property results in an error.
Solution:
Check if the variable is `undefined` or `null` before trying to access its properties.
let person;
if (person) {
console.log(person.name);
} else {
console.log("Person is undefined");
}
You can also use optional chaining (`?.`) to safely access properties:
console.log(person?.name); // Output: undefined (No error thrown)
2. Function Returning `undefined`
A function that doesn't explicitly return a value will implicitly return `undefined`. If you try to use the result of such a function without checking, it can lead to errors.
function getUser() {
// No return statement
}
let user = getUser();
console.log(user.name); // TypeError: Cannot read property 'name' of undefined
Solution:
Always return the expected value from a function, or explicitly handle the `undefined` case.
function getUser() {
return { name: 'Alice' }; // Explicit return
}
let user = getUser();
console.log(user?.name); // Output: Alice
Alternatively, handle the case when `undefined` is returned:
let user = getUser();
if (user) {
console.log(user.name);
} else {
console.log("User is undefined");
}
3. Array Index Out of Bounds
When trying to access an index in an array that doesn't exist, JavaScript returns `undefined`. This can lead to errors if your code assumes the element exists.
Example:
let numbers = [1, 2, 3];
console.log(numbers[3]); // Output: undefined
Solution:
Check the length of the array or ensure the index is within bounds before accessing it.
let numbers = [1, 2, 3];
if (numbers.length > 3) {
console.log(numbers[3]);
} else {
console.log("Index out of bounds");
}
4. Incorrect Variable Scope
JavaScript’s variable scoping can lead to situations where a variable is inadvertently `undefined` due to scope issues. For example, variables declared with `var` inside a function are scoped to that function, while `let` and `const` are block-scoped.
Example:
function greet() {
if (true) {
var greeting = "Hello";
}
console.log(greeting); // Output: Hello (var is function-scoped)
}
function greetAgain() {
if (true) {
let greeting = "Hello";
}
console.log(greeting); // ReferenceError: greeting is not defined
}
Solution:
Be mindful of the scope of your variables and use `let` or `const` instead of `var` to avoid scope-related issues.
function greetAgain() {
let greeting;
if (true) {
greeting = "Hello";
}
console.log(greeting); // Output: Hello
}
5. Misspelled Variable Names
Misspelling variable names can lead to `undefined` references, as JavaScript will treat the typo as a new variable declaration (if not in strict mode) or throw a reference error.
Example:
let userName = "John";
console.log(usrName); // ReferenceError: usrName is not defined
Solution:
Always double-check variable names for typos. Using tools like ESLint or IDE features that highlight undefined variables can help catch these errors early.
How to Handle Undefined or Null Values Gracefully
Handling `undefined` or `null` properly can prevent many common errors in JavaScript. Here are some strategies:
1. Default Parameters
You can set default values for function parameters to avoid `undefined` when no argument is passed.
function greet(name = "Guest") {
console.log(`Hello, ${name}`);
}
greet(); // Output: Hello, Guest
2. Short-circuiting with Logical OR (`||`)
You can use the logical OR (`||`) operator to provide a fallback value if the left-hand side is `undefined` or `null`.
let name;
console.log(name || "Guest"); // Output: Guest
Note: The `||` operator will also short-circuit on falsy values like `0` or `false`. If you want to only check for `null` or `undefined`, use the nullish coalescing operator (`??`):
let name = null;
console.log(name ?? "Guest"); // Output: Guest
3. Optional Chaining (`?.`)
Optional chaining allows you to safely access properties or call methods on `undefined` or `null` objects without throwing an error.
let user = null;
console.log(user?.name); // Output: undefined (No error thrown)
4. Strict Equality Check
Always use strict equality (`===`) when checking for `undefined` or `null` to avoid type coercion errors.
if (user === null) {
console.log("User is null");
}
5. Type Checking
When unsure about the type of a value, use `typeof` to check whether a variable is `undefined`.
if (typeof user !== 'undefined') {
console.log(user.name);
}
Conclusion
Handling `undefined` and `null` references is a crucial part of writing robust JavaScript code. These values often signal the absence of data or a mistake in your logic. By understanding when and why these references occur, and using strategies like optional chaining, default values, and strict equality checks, you can prevent common errors and improve the reliability of your applications.
In summary:
Understand the difference between `undefined` and `null`.
Always check for the presence of values before accessing properties.
Use optional chaining and default values to avoid errors.
Pay attention to scope and variable naming to reduce undefined reference errors.
Comentários