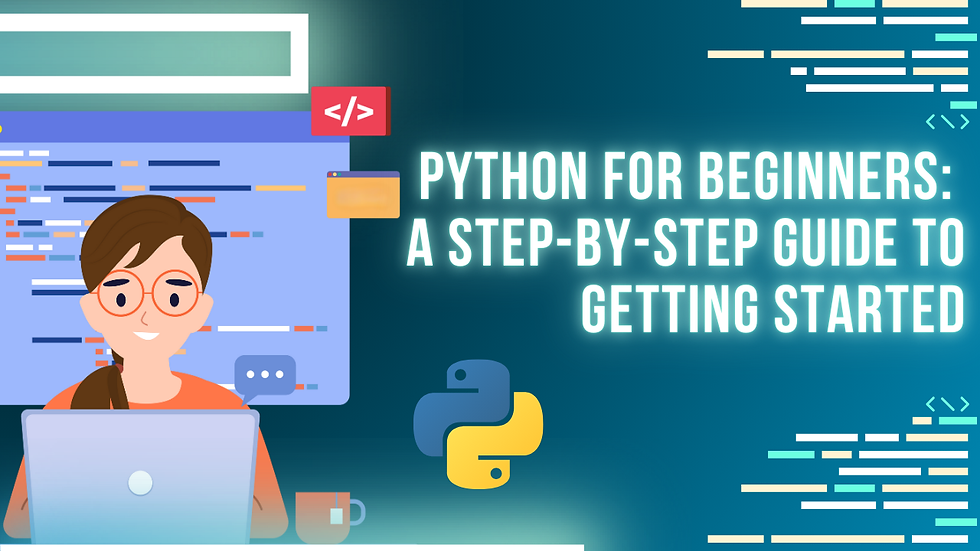
Introduction
Welcome to the world of Python, a powerful and versatile programming language that's perfect for beginners and experienced developers alike. Whether you're looking to dive into web development, data analysis, automation, or just want to learn programming, Python is the language to start with. This guide will walk you through the basics, from setting up your environment to writing your first Python program.
Python for Beginners: A Step-by-Step Guide to Getting Started
Why Python?
Before we dive into the tutorial, let’s understand why Python is such a popular choice:
Easy to Learn: Python has a simple syntax that's similar to English, making it easy to read and write.
Versatile: Python is used in various fields like web development, data science, artificial intelligence, automation, and more.
Community Support: Python has a vast community, meaning there’s plenty of resources, libraries, and frameworks available.
Step 1: Installing Python
To get started with Python, you'll need to install it on your computer. Follow these steps to install Python:
1.1. Download Python
Visit the official Python website at python.org.
Download the latest version of Python for your operating system (Windows, macOS, Linux).
1.2. Install Python
Run the downloaded installer.
Important: Make sure to check the box that says "Add Python to PATH" before clicking the install button. This option will automatically add Python to your system's PATH, making it easier to run Python from the command line.
1.3. Manually Adding Python to the PATH on Windows
If you didn't check the "Add Python to PATH" box during installation, you can add it manually:
Open the Start Menu and search for "Environment Variables."
Select Edit the system environment variables.
In the System Properties window, click on Environment Variables.
In the Environment Variables window, under System variables, find the Path variable, and click Edit.
Click New and add the path to the Python installation directory (e.g., C:\Python39\ or C:\Users\YourUsername\AppData\Local\Programs\Python\Python39\).
Click OK to close all windows.
Now, Python should be accessible from any command prompt window.
Step 2: Setting Up Your Environment
After installing Python, you’ll want to set up a development environment where you can write and run your Python code.
2.1. Using the Python Interactive Shell
Open your terminal or command prompt.
Type python (or python3 on some systems) to start the Python interactive shell.
You can now write and execute Python code directly in the shell.
2.2. Installing an IDE or Text Editor
For a better coding experience, consider using an Integrated Development Environment (IDE) or a text editor:
IDEs: PyCharm, Visual Studio Code, Spyder.
Text Editors: Sublime Text, Atom, Notepad++.
Step 3: Writing Your First Python Program
Let’s start by writing a simple Python program to get a feel for the language.
3.1. The "Hello, World!" Program
Open your text editor or IDE.
Type the following code:
python
print("Hello, World!")
Save the file with a .py extension, for example, hello_world.py.
Run the program:
In the terminal, navigate to the directory where your file is saved.
Type python hello_world.py (or python3 hello_world.py).
You should see the output: Hello, World!
Step 4: Understanding Basic Python Concepts
Now that you’ve written your first program, let’s explore some basic Python concepts.
4.1. Variables and Data Types
Variables: Think of variables as containers for storing data. Python doesn’t require you to declare the data type.
name = "Alice" age = 25
Data Types:
Strings: "Hello"
Integers: 42
Floats: 3.14
Booleans: True, False
4.2. Basic Operations
Arithmetic: Python supports basic arithmetic operations like addition, subtraction, multiplication, and division.
result = 10 + 5 # result is 15
String Operations:
greeting = "Hello, " + "World!"
Step 5: Control Flow (If Statements, Loops)
Control flow statements allow you to execute code based on conditions or repeat code.
5.1. If Statements
Syntax:
if age > 18: print("You are an adult.") else: print("You are a minor.")
5.2. Loops
For Loop:
for i in range(5): print(i)
While Loop:
count = 0 while count < 5: print(count) count += 1
Step 6: Functions
Functions allow you to write reusable blocks of code.
6.1. Defining a Function
Syntax:
def greet(name): return "Hello, " + name
Calling a Function:
print(greet("Alice"))
Step 7: Working with Lists
Lists are used to store multiple items in a single variable.
7.1. Creating a List
Example:
fruits = ["apple", "banana", "cherry"]
7.2. Accessing List Items
Indexing:
print(fruits[0]) # Output: apple
7.3. Adding and Removing Items
Add:
fruits.append("orange")
Remove:
fruits.remove("banana")
Step 8: Reading and Writing Files
Learn how to work with files in Python.
8.1. Reading a File
Example:
with open("example.txt", "r") as file: content = file.read() print(content)
8.2. Writing to a File
Example:
with open("example.txt", "w") as file: file.write("Hello, World!")
Step 9: Handling Errors with Try/Except
Learn how to handle errors and exceptions in Python.
9.1. Basic Error Handling
Example:
try: result = 10 / 0 except ZeroDivisionError: print("Cannot divide by zero!")
Step 10: Where to Go Next?
You’ve covered the basics! Here are some ideas on what to learn next:
Object-Oriented Programming (OOP): Learn how to create classes and objects.
Libraries and Frameworks: Explore popular libraries like pandas, numpy, and frameworks like Django and Flask.
Projects: Start a small project like a to-do list app, a simple web scraper, or a game.
Congratulations on completing your first steps in Python! This guide covered the fundamentals, and you’re now ready to explore more advanced topics.
Commentaires